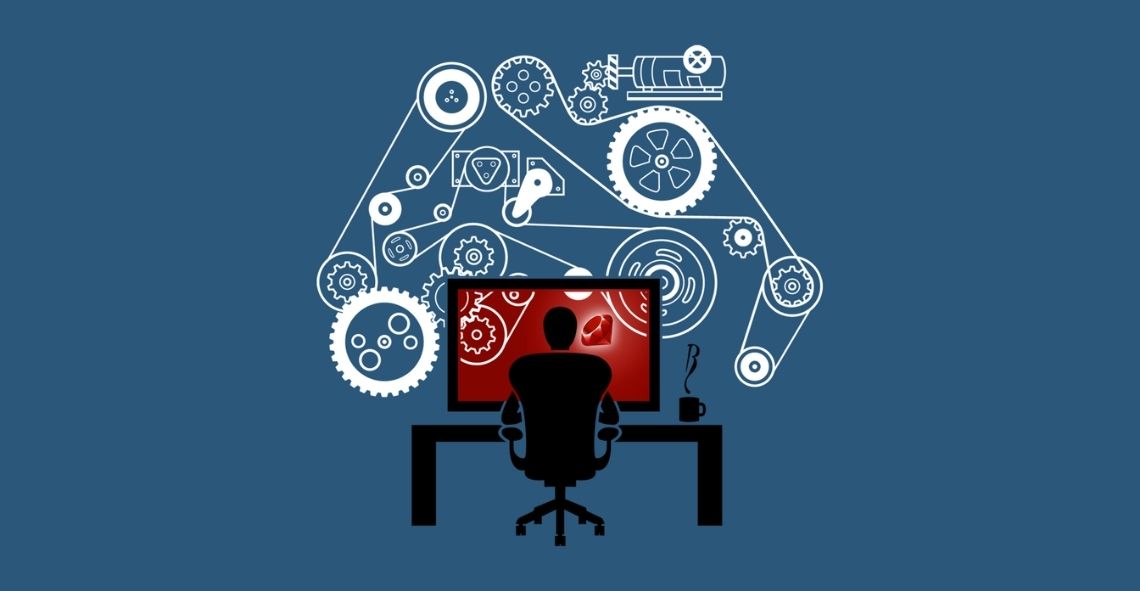
Once the environment is configured, we are ready to run programs.
Let’s create the appropriate Hello World in a helloworld.rb file which will contain
puts "Hello World !!!"
and then we launch
ruby ./ helloworld . rb
The output we get will be
Hello World !!!
The reduction of the code necessary to obtain the result is very considerable!
Let’s code
Let’s write our first class.
class Automobile def initialize ( make , model ) @brand = make @model = model end end
Let’s create an instance of our first class
automobile = Automobile . new ( "Honda" , "Jazz" )
Let’s add a first method to the class
def dial_name "Auto: # @ make - # @ model" end
Then we invoke the method
car_name = ( car . dial_name )
and then let’s send it to the screen
puts ( auto_name )
The result will be:
Car : Honda - Jazz
Naming Convention
As can be seen from the first lines of code shown, we note that the class name begins with a capital letter (Automobile).
We also note that the body of the method is defined by the keyword
def
followed by the method name and ending with
end
A variable preceded by @ is an instance variable.
@variable_instance
For a class variable we would have had @@ followed by the variable name
@ @Variabile_di_ class
For a global variable we would have had
$ globalvariable
or
$ GLOBAL_VARIABLE
The # symbol precedes the comment. Even if within a string # [expression] is replaced by [expression] as in the example above in the compose_name method.
Let’s extend the class
Let’s create a class that extends Automobile.
class Utility car < Automobile def dial_name super + "what a utility vehicle !!!!" end end
Then we add:
automobile = Economy car . new ( "Honda" , "Jazz" ) car_name = ( car . dial_name ) puts ( car_name )
The result will be:
Car : Honda - Jazz what a small car !!!!
A few lines of sample code
We introduce the first control structure. The aim is to be able to move easily in the situations that we will encounter below.
Control structures, combined with Iterators and Blocks, are “core concepts” in Ruby. It should be noted that the continuous use of the language will lead us more and more to a “Rubyst” approach that will distance us from a classic implementation mode.
In the Automobile class we add the define_value method with the level parameter (we use the notation without paranthesis).
def define_value level @level = "" if level == 0 @level = "Poor" else @level = "Good" end end
Then we invoke the method
livello_auto = ( Car . definisci_valore ( 3 ))
3.times { puts ( livello_auto )}
The output will obviously be
Good
Good
Good
We highlight that we could have written the content of the method as
if l == 0 : @level = "Poor" else @level = "Good" end
or
@level = if l == 0 then "Poor" else "Good" end
Try and enjoy !!!
We have never explicitly specified a “return value”. We could do it if needed, otherwise the last variable set in the method will be returned. This is very convenient and not to be forgotten.
Let’s move on to a slightly more complex example.
Taking a leap from automobiles to the animal kingdom, we create a full class of its public attributes and accessories.
class Animal def initialize ( name , class ) @name = name @class = class end def name return @name end def class return @class end end
We could have used a more streamlined notation for accessories.
class Animal def initialize ( name , class ) @ name = name @Class = class end attr_reader : name ,: class end
This allows you to quickly list all the attributes you need to access in reading (attr_reader) or writing (attr_writer) simply by reporting them separated by commas as shown above.
Let’s write a few lines of purely didactic code. TO??? our intention is to read the contents of a file, scroll through it, introduce the Array class, write examples of loops and string operations. If we then intend to extend or better understand our code, we can refer to the RDoc documentation of the basic installation to get some ideas about it.
We prepare a “filetoread” text file with the following content (or with content that has the same formatting)
Alligator Reptile Hawk Bird Lion Mammal Fly Insect
Then we create the file run.rb with the following content
require ' animal ' # Declare an Array @animals = Array . new # We loop through the elements of the file 'filetoread' File . open ( "filetoread" ) do | element | # Scroll through each line of the element file . each do | row | # The first element of the line is the name, # the second the animal class. # We use regular expressions in order to # separate the two strings name , class =
row . chomp . split ( / s * s * / ) # Instantiate an Animal animal = Animal . new ( name , class ) # Let's add the elements to the @animals array . push ( animal ) # We do this again with a different notation @animals << animal end end # We operate on the Array and reverse @animals = @animals . reverse # Let's create a file to write f =
File . new ( "filetowrite" , "w" ) # Take each Animal element # and write name and class to the @animals file . each { | animal | f . syswrite ( animal . class + "" + animal . name + "
" )
}
We run ruby ./execute.rb.
Let’s see the contents of the file created “filetowrite”. It should contain
Insect Fly Insect Fly Mammal Lion Mammal Lion Bird Hawk Bird Hawk Reptile Alligator Reptile Alligator
If we analyze the written code, also supported by the comments, we have introduced a series of expressions and concepts not mentioned previously.
We used the keyword require that allows you to import references to external classes, the Array class and the syntax @ array.each {| arrayelement | … do something on each element …}, operations on the String class (also using Regular Expression), the use of the File class used to read and write files
Simple … and fun.
We therefore invite you to download the attachment “ruby-1.zip” from the menu at the top left.
Conclusions
We did a quick overview of where Ruby ranks, particularly as seen by a Java Programmer. We moved on to installing the environment and “running” our first lines of code starting to understand the ease of use. We are therefore ready to deepen the language. We just have to wait … for the next article.